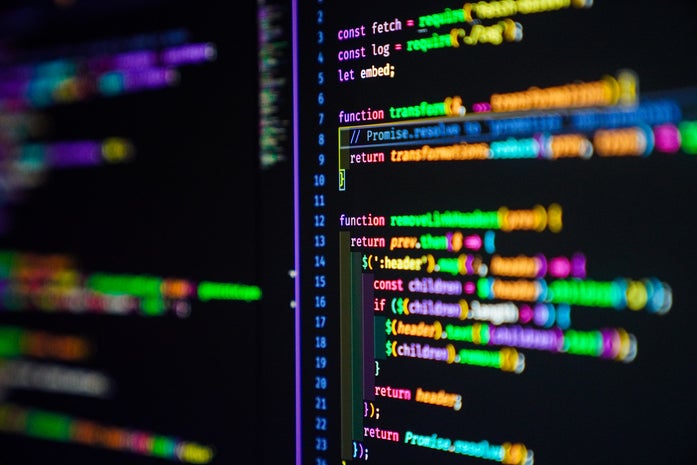
This blog does not specifically discuss a blockchain project, crypto or part of a blockchain, such as the consensus mechanism. In this blog, I provide a simplified tutorial to create a smart contract code on the Ethereum blockchain, via the Ethereum Virtual Machine.
Don't worry, I will explain the concepts that come along so that even if you have never coded before, you can follow this as well.
The goal of this blog
This blog is primarily intended for new developers who want to get started with Ethereum. It will use Solidity, Ethereum's programming language. With this, we will build our first smart contract.
What is a smart contract?
The term smart contract was coined by Nick szabo in 1997. Smart contracts are nothing more but programs that exist on the blockchain. These "smart contracts" can be used by external method calls or calls to other smart contracts. These smart contracts are executed in the EVM (Ethereum virtual machine). Smart contracts can reduce malicious intents, fraudulent activities and the need for a trusted intermediary when properly written and verified.
What is Solidity?
Smart contracts are nothing but programs and you need a programming language to write these programs. Ethereum's core contributors invented a programming language called Solidity to write smart contracts (computer programs that run on the blockchain). Solidity is a language inspired by JavaScript, C++ and Python. It has a syntax similar to JavaScript. Solidity is not the only language you can use to write smart contracts. There are other languages that can be used to write smart contracts too, such as Vyper, the most popular and widely used smart contract language after Solidity.
Our first smart contract
Our contract will allow us to store an unsigned integer (uint) and retrieve it.
1. // SPDX-License-Identifier: MIT
2. pragma solidity >=0.4.0 <0.7.0;
3. contract SimpleStorage {
4. uint storedData;
5. function set(uint x) public {
6. storedData = x;
7. }
8. function get() public view returns (uint) {
9. return storedData;
10. }
11. }
Line 1: Specify SPDX license type. When the source code of a smart contract is made available to the public, these licenses can help resolve/avoid copyright issues. If you do not want to specify a license type, you can use a special value UNLICENSED or just skip the whole comment (that does not produce an error, just a warning).
Line 2: On the first line we are letting the program know which Solidity compiler we want to use. For instance, we are using any version between ≥ 0.4.0 and <0.7.0 .
Line 3: We are declaring and naming our code as Simplestorage. It is normal to use the same filename as the contract name. For example - this contract will be saved in the file name SimpleStorage.sol (.sol is the file extension for solidity smart contracts, like JPEG for pictures).
Line 4: We are declaring a uint (Unsigned Integer) variable named storedData, this variable will be used to store data.
Line 5-7: We will add a set function, using which we will change the value of our variable storeData. Here set function is accepting a parameter x whose value, we are storing into storeData. In addition, the function is marked as public which means that the function can be called by anyone.
Line 8-10: We will add a get function to retrieve the value of storeData variable. This function is marked as view which tells Solidity compiler that this is a read-only function.
Getting confused? Let's explain a few terms used here:
uint:
-
Unsigned Integers (often called "uints") are just like integers (whole numbers) but have the property that they don't have a + or - sign associated with them. Thus they are always non-negative (zero or positive). We use uint's when we know the value we are counting will always be non-negative. For example, if we are counting the number of players in a game, we could use a uint because there will always be 0 or more players. The same applies to storing data: there will always be 0 or more transactions.
Uint stored data
- In short, this means that the store data is always nonnegative, either 0 or higher. We use a uint in this situation in combination with the stored data because we know that the value of the stored data will always be positive. We can't have a negative value.
set:
-
A function is a group of reusable code which can be called anywhere in your program. This eliminates the need of writing the same code again and again. It helps programmers in writing modular codes. Functions allow a programmer to divide a big program into a number of small and manageable functions.
- A function is a block of organized and reusable code that performs an action. Functions allow a programmer to manage code that is modular and reusable. Different programming languages name these blocks of organized code functions, methods, sub-routines, procedures, etc. If you come across these terms just remember that it is the same concept.
get
- This speaks for itself actually. We are going to retrieve the value of the storeData variable.
}
- Curly braces separate a block of code. Use braces to wrap code. It might not always be necessary, but it is a best practice that will make your code readable, and more reliable.
Deploying the smart contract
After writing a smart contract it must be deployed to the Ethereum network, we will deploy our smart contract using Remix. There are other ways to deploy smart contracts, but to make it beginner-friendly, we will use Remix. Remix is an online web Ethereum IDE. It is simple and supports many features. So open Remix through this link.
I will not elaborate on how the smart contract code can be used in the Remix tool. I will elaborate on that next time. The purpose of this blog was to give insight into what a programming code on the Ethereum blockchain looks like. By doing so, I hope you got a sense of how smart contracts are created in general.
Reactie plaatsen
Reacties