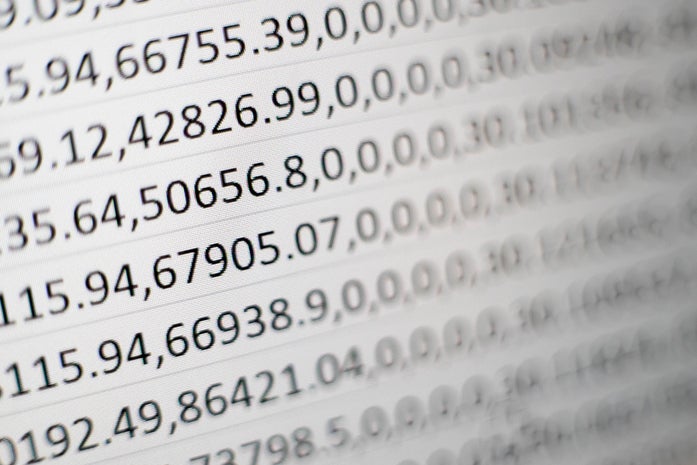
Ever wonder what a blockchain looks like? Wondering what a blockchain code looks like? Then you've come to the right place! In this blog, I will outline what a blockchain actually looks like. In doing so, I will also provide a piece of code that you can use yourself when building your first blockchain network!
What is a blockchain?
A blockchain is a decentralized, distributed ledger that records transactions on multiple computers. It is often used to secure digital currencies like Bitcoin, but it can also be used to record other types of data. In this blog, we will explore how to code a simple blockchain using Python.
Step 1: Create a Block
The first step in building a blockchain is to create a block. A block is a container for transactions and has the following components:
- A unique identifier (hash)
- The previous block's hash
- A timestamp
- Data (e.g. transactions)
Here is an example of a simple block in Python:
import hashlib
import time
class Block:
def __init__(self, data, previous_hash):
self.timestamp = time.time()
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def calculate_hash(self):
data = str(self.timestamp) + str(self.data) + str(self.previous_hash)
return hashlib.sha256(data.encode()).hexdigest()
In the __init__ method, we initialize the block with a timestamp, data, and the previous block's hash. We also calculate the hash of the current block using the calculate_hash method. This method concatenates the timestamp, data, and previous hash and then calculates the SHA-256 hash of the resulting string.
Step 2: Create a Blockchain
Now that we have a block class, we can create a blockchain by linking together a series of blocks. Here is an example of a simple blockchain class in Python:
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block("Genesis Block", "0")
def get_latest_block(self):
return self.chain[-1]
def add_block(self, new_block):
new_block.previous_hash = self.get_latest_block().hash
new_block.hash = new_block.calculate_hash()
self.chain.append(new_block)
In the __init__ method, we create a blockchain with a single "genesis" block. The genesis block is the first block in the chain and serves as a reference point for all future blocks.
The get_latest_block method returns the most recent block in the chain. The add_block method adds a new block to the chain by setting the new block's previous hash to the hash of the latest block and then calculating the new block's hash.
Step 3: Test the Blockchain
Now that we have a block and a blockchain class, we can test our implementation by creating a new blockchain and adding some blocks to it.
blockchain = Blockchain()
blockchain.add_block(Block("Block 1", ""))
blockchain.add_block(Block("Block 2", ""))
blockchain.add_block(Block("Block 3", ""))
for block in blockchain.chain:
print("Timestamp:", block.timestamp)
print("Data:", block.data)
How to execute the blockchain code?
To execute the blockchain code, you will need to have Python installed on your computer. You can then create a Python file (e.g. blockchain.py) and copy the code into it.
Once you have the code in a Python file, you can run it from the command line using the following command:
python blockchain.py
This will execute the code and you should see the output of the test blockchain that we created earlier, which includes the timestamps and data for each block in the chain.
You can also run the code in an interactive Python shell by opening a terminal and typing Python. This will bring you into the Python interpreter where you can enter the code line by line.
Example:
$ python
>>> from blockchain import Block, Blockchain
>>> blockchain = Blockchain()
>>> blockchain.add_block(Block("Block 1", ""))
>>> blockchain.add_block(Block("Block 2", ""))
>>> blockchain.add_block(Block("Block 3", ""))
>>> for block in blockchain.chain:
... print("Timestamp:", block.timestamp)
... print("Data:", block.data)
...
Timestamp: 1623479823.9847298
Data: Genesis Block
Timestamp: 1623479824.9847298
Data: Block 1
Timestamp: 1623479825.9847298
Data: Block 2
Timestamp: 1623479826.9847298
Data: Block 3
Reactie plaatsen
Reacties